Arrays are an essential part of programming, as they allow us to store and manipulate multiple values of the same data type under a single variable name. In this blog, we’ll take a closer look at arrays in C# and explore some examples to help you understand their practical use.
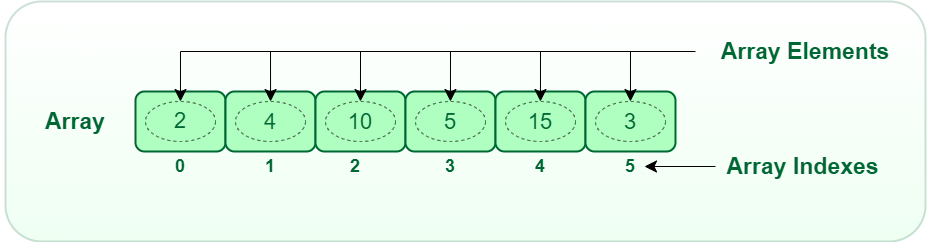
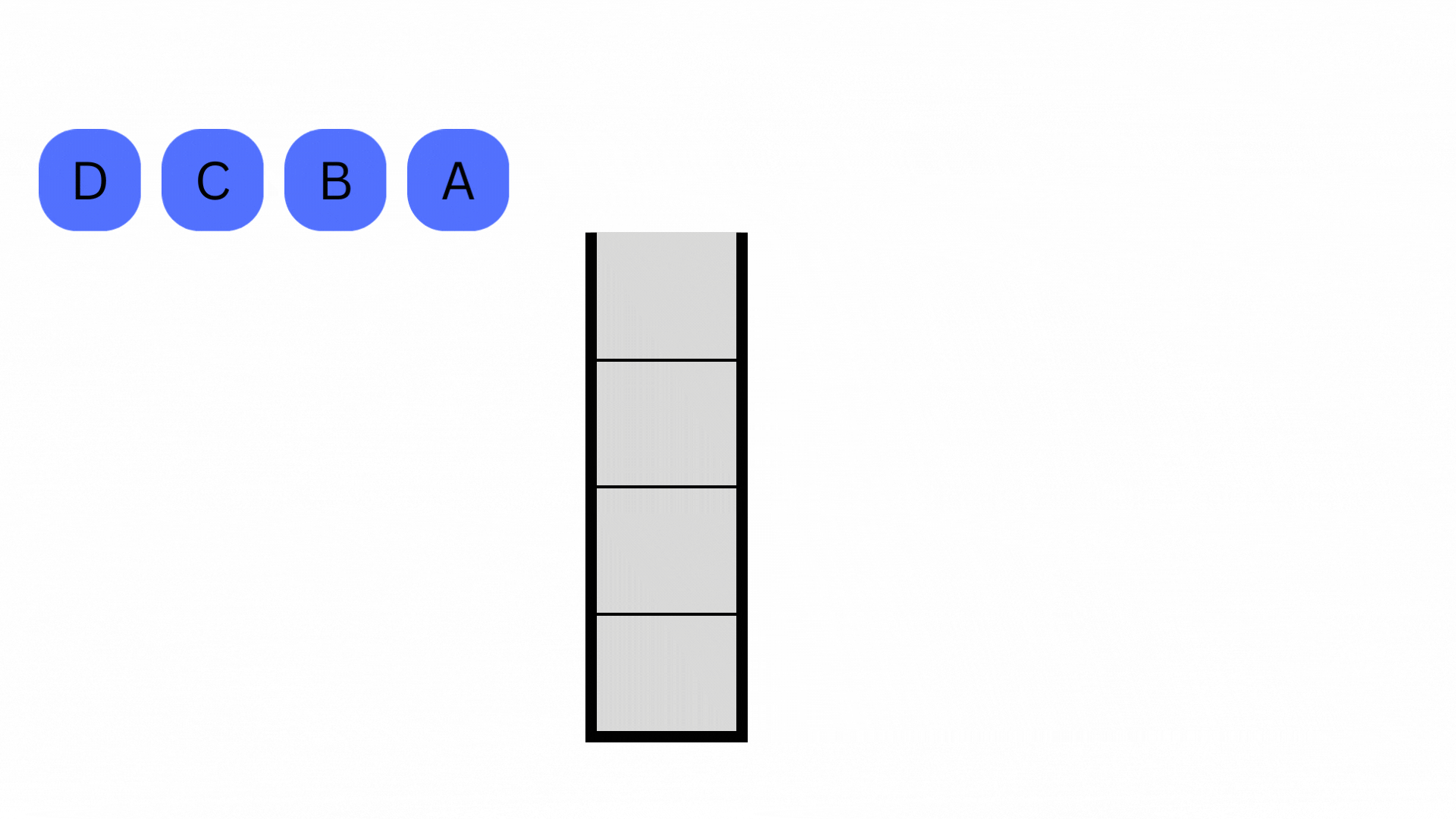
Declaring an array in C#
In C#, arrays are declared by specifying the data type followed by square brackets [] and then the variable name. For example, the following code creates an integer array named “numbers” that can hold five integer values:
int[] numbers = new int[5];
Here, we are declaring an integer array with five elements. We specify the data type (int), followed by square brackets [] to indicate that this is an array. The keyword “new” is used to allocate memory for the array, and the value in the brackets (5) represents the number of elements that the array can hold.
Initializing an array
Once we have declared an array, we can initialize it by assigning values to each of its elements. There are a few different ways to do this in C#.
1) Initialize array elements during declaration:
int[] numbers = new int[] {1, 2, 3, 4, 5};
Here, we are initializing the array with five integer values: 1, 2, 3, 4, and 5.
2) Initialize array elements without declaring size:
int[] numbers = {1, 2, 3, 4, 5};
In this case, the compiler will automatically determine the size of the array based on the number of elements that are provided in the initialization.
Accessing array elements
To access the elements of an array, we use the array index. Array indexing in C# starts at zero. For example, to access the first element of the “numbers” array, we use the following code:
int firstNumber = numbers[0];
Here, we are using the array index (0) to access the first element of the “numbers” array and store its value in the “firstNumber” variable.
Looping through an array
Loops are a powerful tool when it comes to working with arrays. For example, the following code uses a “for” loop to iterate through each element of the “numbers” array and print its value to the console:
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
Here, we are using a “for” loop to iterate through each element of the “numbers” array. We are using the “Length” property to determine the number of elements in the array. The loop starts at 0 and continues until the index is equal to the length of the array minus one.
Conclusion
Arrays are an essential part of programming, and they allow us to store and manipulate multiple values of the same data type under a single variable name. In C#, arrays are declared by specifying the data type followed by square brackets [] and then the variable name. We can initialize arrays using various techniques and access their elements using the array index. Loops are an excellent tool to work with arrays, allowing us to iterate through each element and perform operations on them.
The fact that you like your website is clear; nonetheless, a number of your posts may use some editing to improve their spelling and punctuation. I will most certainly come back; but, the fact that so many of them contain misspellings is incredibly annoying to me. On the one hand, I will absolutely come back.
Thank you I have just been searching for information approximately this topic for a while and yours is the best I have found out so far However what in regards to the bottom line Are you certain concerning the supply
I very delighted to find this internet site on bing, just what I was searching for as well saved to fav
Hi there to all, for the reason that I am genuinely keen of reading this website’s post to be updated on a regular basis. It carries pleasant stuff.
you are in reality a just right webmaster The site loading velocity is incredible It seems that you are doing any unique trick In addition The contents are masterwork you have performed a wonderful task on this topic
I do trust all the ideas youve presented in your post They are really convincing and will definitely work Nonetheless the posts are too short for newbies May just you please lengthen them a bit from next time Thank you for the post
Hello my loved one I want to say that this post is amazing great written and include almost all significant infos I would like to look extra posts like this